Explain Javascript map function like I’m five
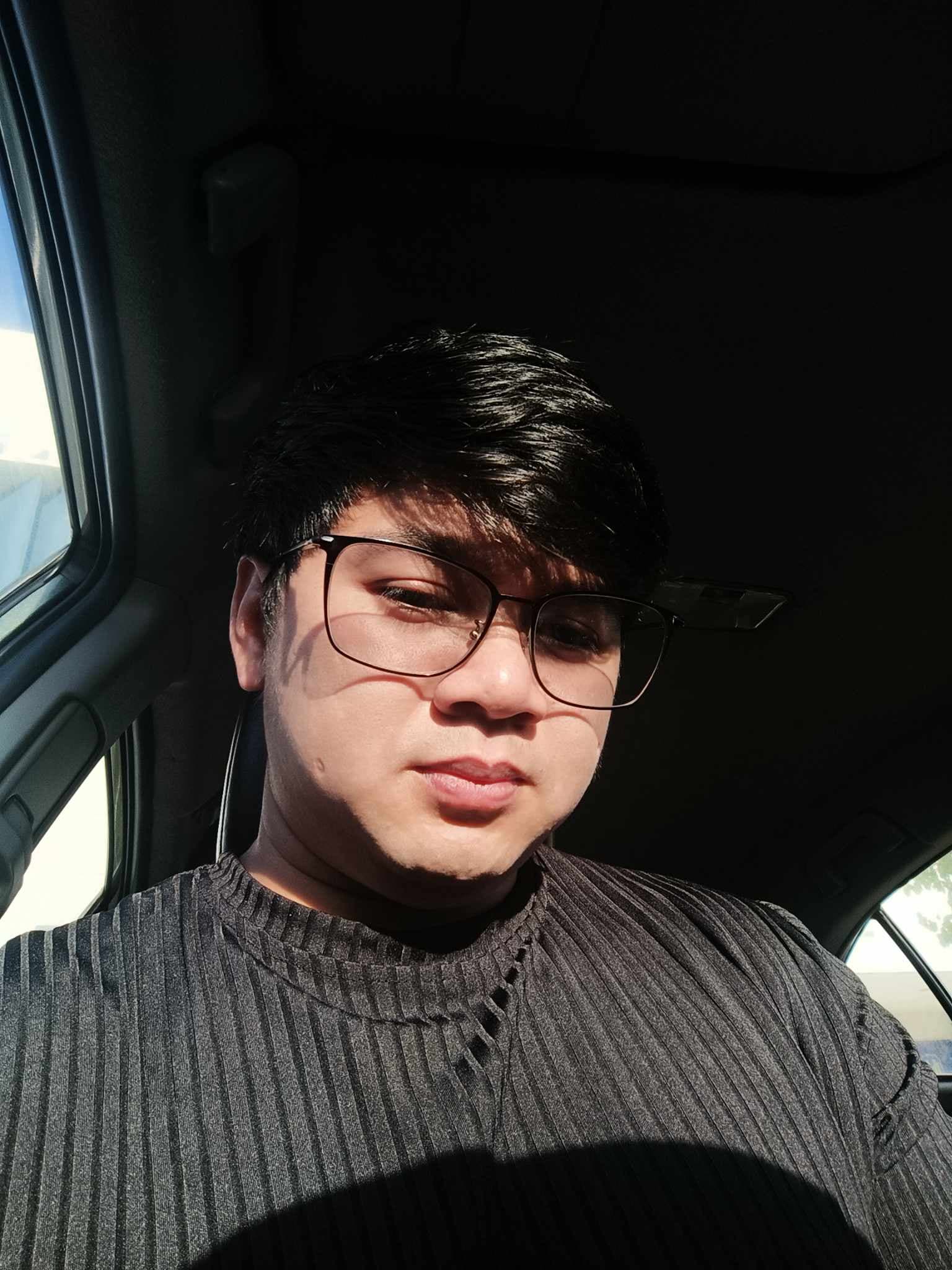
Jomar M
The Map()
function in JavaScript is a way to create a special kind of data structure called a "map". A map is like a list or an array, but instead of using numbers to access the data inside, you use special keys.
For example, let's say you have a list of colors and you want to access the color red. With a regular array, you would have to remember that red is the first item in the list, so you would use the number 0
to access it. With a map, you can give the color red a special key, like "favoriteColor", and then use that key to access the color red.
Here's an example of how to create a map and add data to it using the Map()
function:
// create a new map let colors = new Map(); // add some colors to the map colors.set("favoriteColor", "red"); colors.set("leastFavoriteColor", "orange"); colors.set("neutralColor", "gray");
Now that we have some data in our map, we can access it using the keys we set. Here's how we would access the color red from our example:
let favoriteColor = colors.get("favoriteColor"); // favoriteColor = "red"
Notice that we used the get()
function to access the data in our map. This is how you access data in a map. You use the get()
function and pass in the key you want to use to access the data.
Maps are useful in a variety of situations. For example, if you have a lot of data and you want to organize it in a way that's easy to access and manage, using a map can be a great solution. Maps are also useful when you have data that doesn't have a natural order, like a collection of user objects where each user has a unique ID.
Here's an example of how you could use a map to store and access user data:
let users = new Map(); // add some users to the map users.set(1, {name: "Alice", age: 25}); users.set(2, {name: "Bob", age: 30}); users.set(3, {name: "Carol", age: 35}); // access a user's data let user = users.get(2); // user = {name: "Bob", age: 30}
In this example, we created a map called users
and added three user objects to it. We then used the get()
function to access the user with the ID 2
. This is just one example of how you can use a map to store and access data in JavaScript.
Overall, the Map()
function is a useful tool for creating and working with maps in JavaScript. It allows you to create a data structure that allows you to access data using keys instead of numbers, making it easier to organize and manage your data.
Related Posts

Custom Website Development Cost vs. WordPress: Making the Right Choice
Choosing between WordPress and custom website development can be a daunting task. This article provides a detailed comparison of both platforms, considering factors like cost, design, functionality, scalability, and security, to help you make an informed decision for your business.

Best Headless CMS in 2025: Your Guide to Choosing the Right Platform
The future of web development is headless, and the market is booming. By 2025, the global headless CMS market is expected to reach $3.9 billion. With so many powerful platforms vying for your attention, choosing the best headless CMS for your needs can be overwhelming

Frontend for Headless CMS: A Comprehensive Guide
Discover how to build an efficient frontend for a headless CMS with this comprehensive guide. Learn the best practices, tools, and techniques to create dynamic, scalable websites that separate content management from presentation for enhanced flexibility and performance.