How to Check if Third-Party Cookies Are Enabled in the Browser
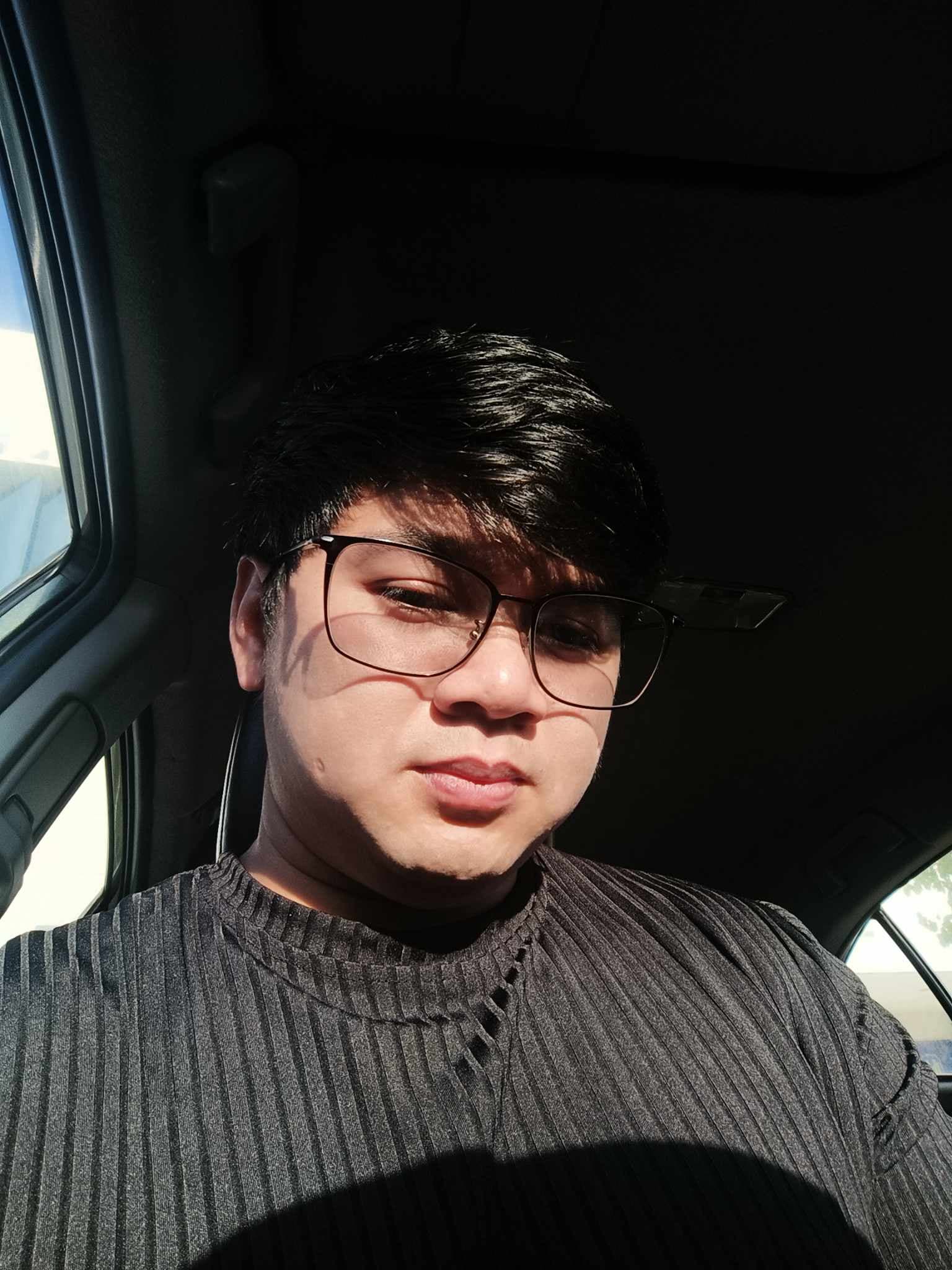
Jomar M
Today I learned how to check if third-party cookies are enabled in a browser using a simple JavaScript approach. Third-party cookies are often blocked by browsers due to privacy concerns, and if your web app relies on them for certain features like cross-site tracking or integrations, it’s essential to verify if they are supported by the user’s browser.
Here’s a code snippet that checks for third-party cookie support using an iframe and postMessage. This method sends a message from a third-party domain to the parent window, allowing us to detect if cookies from that domain are accessible.
import { useState, useEffect } from "react"; export const useThirdPartyCookieCheck = () => { const [isSupported, setIsSupported] = useState(false); useEffect(() => { const frame = document.createElement("iframe"); frame.id = "3pc"; frame.src = "https://chamithrepo.github.io/create-third-party-cookie/"; //Add your hosted domain URL here frame.style.display = "none"; frame.style.position = "fixed"; document.body.appendChild(frame); window.addEventListener( "message", function listen(event) { if (event.data === "3pcSupported" || event.data === "3pcUnsupported") { setIsSupported(event.data === "3pcSupported"); document.body.removeChild(frame); window.removeEventListener("message", listen); } }, false ); }, []); return isSupported; };
Call the hook within your react component
const thirdPartyEnabled = useThirdPartyCookieCheck()
Related Posts

The Undeniable Advantages of Custom Website Development
The Undeniable Advantages of Custom Website Development

How Next.js Partial Prerendering Works: A Deep Dive
Next.js Partial Prerendering (PPR) merges static and dynamic rendering by leveraging React Suspense to separate dynamic components and enabling static prerendering of the rest.

Custom Website Development Cost vs. WordPress: Making the Right Choice
Choosing between WordPress and custom website development can be a daunting task. This article provides a detailed comparison of both platforms, considering factors like cost, design, functionality, scalability, and security, to help you make an informed decision for your business.