What’s the difference between typescript null and undefined?
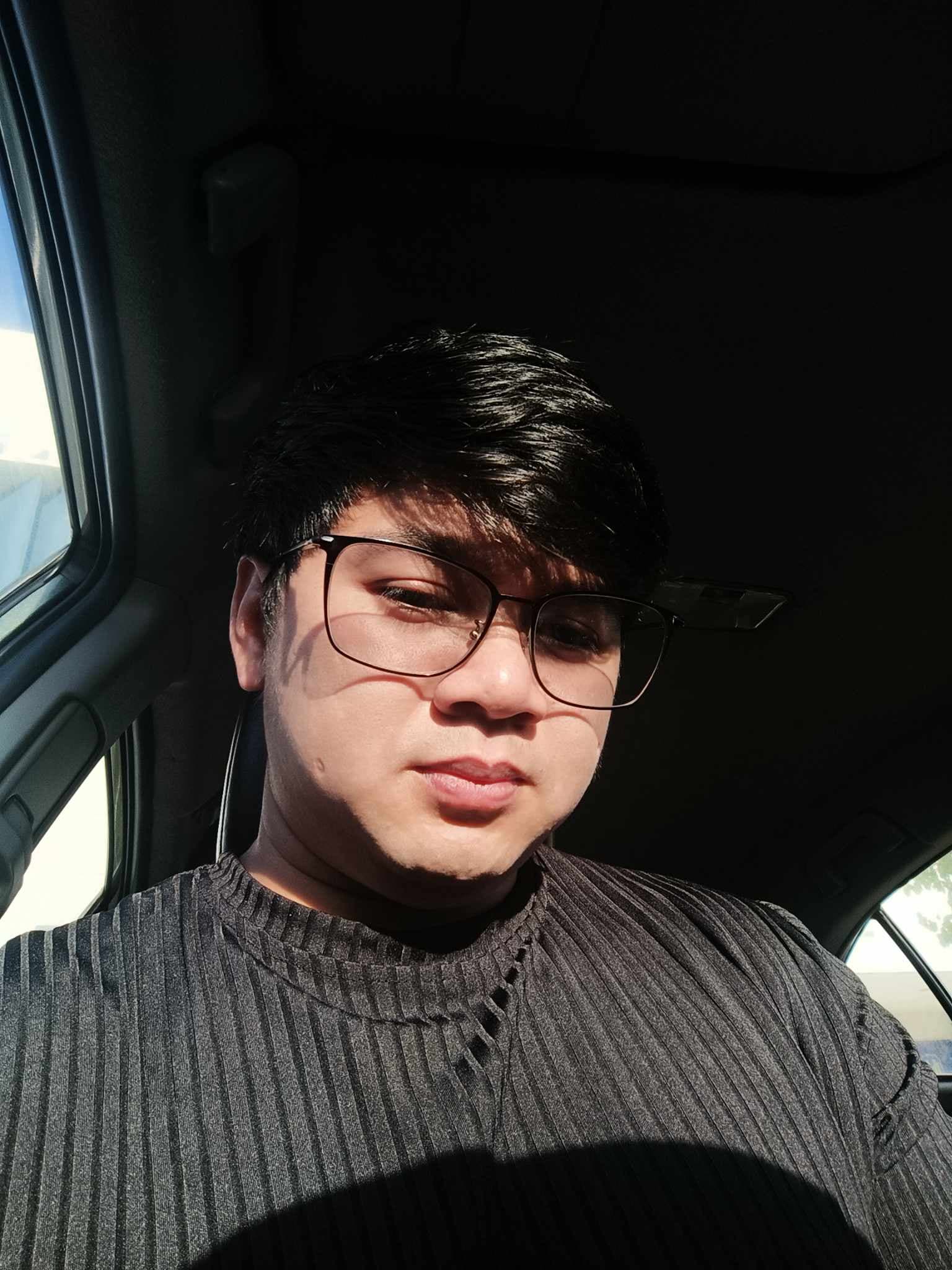
Jomar M
Today I learned about the distinction between null and undefined in TypeScript—two terms that often confuse developers but serve different purposes.
Key Points:
-
null: Represents the intentional absence of any value. Use it when you want to specify that a variable has no value on purpose.
-
undefined: Indicates that a variable hasn’t been assigned a value yet. It’s the default state for uninitialized variables.
Why It Matters:
• Using null helps to clearly communicate intentional absence, making your code easier to understand.
• Leveraging undefined for uninitialized values is useful when you expect the variable to be assigned later.
This distinction avoids unnecessary bugs and keeps the codebase predictable.
Example of null
let user = null; // Intentional absence of a value console.log(user); // Output: null
Example of undefined
let user; // Variable declared but not initialized console.log(user); // Output: undefined
Checking for both
function checkValue(value: any): void { if (value === null) { console.log("Value is null: Intentionally absent"); } else if (value === undefined) { console.log("Value is undefined: Not yet initialized"); } else { console.log("Value exists:", value); } } checkValue(null); // "Value is null: Intentionally absent" checkValue(undefined); // "Value is undefined: Not yet initialized" checkValue("Jomar"); // "Value exists: Jomar"
Related Posts

The Undeniable Advantages of Custom Website Development
The Undeniable Advantages of Custom Website Development

Custom Website Development Cost vs. WordPress: Making the Right Choice
Choosing between WordPress and custom website development can be a daunting task. This article provides a detailed comparison of both platforms, considering factors like cost, design, functionality, scalability, and security, to help you make an informed decision for your business.

Best Headless CMS in 2025: Your Guide to Choosing the Right Platform
The future of web development is headless, and the market is booming. By 2025, the global headless CMS market is expected to reach $3.9 billion. With so many powerful platforms vying for your attention, choosing the best headless CMS for your needs can be overwhelming